Web development is a changing entity in the technology world with ever-changing trends and frequently emerging new technologies. At the same time, it is constant with rules and standards to follow. A bit controversial, isn’t it? The explanation is simple – even though the emphasis might shift from one technology to another, there are the best practices web development always employs as they are time-tested and proven to be efficient.
In this article, we’ll look into the most appreciated and useful techniques and strategies that professional web developers like and utilize for outstanding results.
Best web development practices
In general, web development is a complex process with tons of technologies and tactics. It is already hard to learn web development as it is taking into account the abundance of languages, frameworks, and rules. If you don’t know where to start, opt for learning and applying web development best practices. Here, our specialists share the best front-end and back-end directives they personally adhere to.
Front-end development best practices for web
In this section, we’ll explore the most useful practices of basic front-end development that will help you facilitate both programming and styling processes. To make it clearer, we divided them by fundamental technologies – HTML, CSS, and JavaScript.
HTML
- Start with a
<!DOCTYPE>
declaration. It's crucial to inform browsers about the version of the HTML document is written in. This simple<!DOCTYPE html>
instruction in HTML5 is required to ensure standard mode rendering of your pages. Otherwise, you’ll force browsers to render pages in Quirks mode.
- Single H1 on page. It’s a W3C Specification official recommendation for developers to use only one H1 on a page. Including several first-level heading tags will impair the search engine performance of your pages.
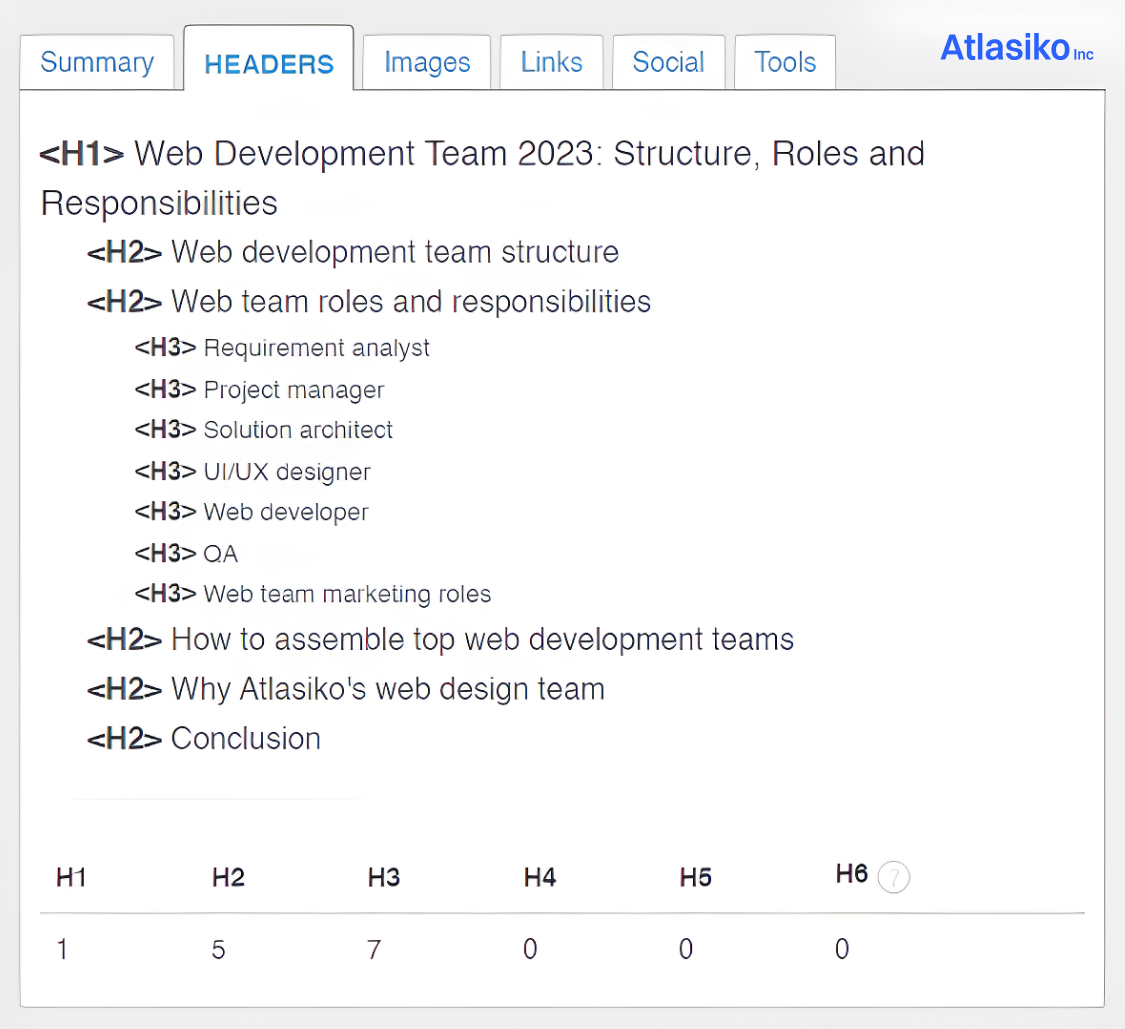
- Alt attribute for images. Don’t forget to specify the
alt
attribute in your<img />
tags. They have to describe precisely what the image is about since this description will be shown in case the image fails to load. This element can have a significant impact on the accessibility qualities of your web pages as well as search engine rankings. - Use HTML validator. Usually, professionals don’t need to validate their HTML code but in some more complicated cases, you just need to make sure it’s correct. Think twice, cut once. Check your code with HTML validators if needed. W3C Markup Validation Service is a great option to use for validation.
- Use semantic markup. Our best practices in the website development blog has already brought to light the importance of semantic markup in HTML5. In short, the utilization of semantic tags makes your web pages structured and more comprehensible for both devs and search engines.
<!-- BAD APPROACH -->
<div id="header">
<a href="https://atlasiko.com/">Atlasiko Home</a>
</div>
<div id="article">
<p>Content</p>
</div>
<!-- GOOD APPROACH -->
<header>
<a href="https://atlasiko.com/">Atlasiko Home</a>
</header>
<article>
<p>Content</p>
</article>
- Close your tags. Even though browsers will be able to run your HTML code anyway, it’s still considered a bad practice to leave tags unclosed. Just like with undeclared
<!DOCTYPE>
, unclosed tags can cause errors in web page rendering. Some programmers rely on in-build validators to close their tags but they can put closing tags in the wrong places in the code causing web page crashes. Make closing tags your good habit as one of the best web development practices. - Write code in lowercase. In this situation, writing in code lowercase isn’t a matter of browser or bot parsing but of maintainability. Browsers can execute your code regardless but for other devs, who also work with your HTML documents, it’s easier to read and maintain code in lowercase.
CSS
- Import as few stylesheets as possible. Every additional CSS file negatively impacts the critical rendering path (CRP). The more stylesheets or stylesheet requests are included in your HTML document, the more time browsers will need to build the CSS objective model (CSSOM). To optimize your CRP and overall performance, opt for essential-only styles to represent your content.
- Don’t use inline styles. Out of three methods of adding CSS (external, internal, and inline), web developers consider the use of inline styles not just a bad but appalling practice. Adding different style rules to each element can turn the development process, web page organization and performance into a nightmare. Inline CSS impedes many important principles of web development such as consistency, maintainability, and accessibility.
- Don’t use
!important
property. This rule overrides all previous styles in a particular element unless there’s another!important
rule for each specific property. Web developers recommend abstaining from using it unless there’s no other way to add specificity. Otherwise, comprehending and debugging your CSS code will be rather complicated.
- Use shorthand properties. They allow you to set the values of several other CSS properties at once. You can save time and effort by writing style sheets that are more clean and readable by utilizing a shorthand property.
<!-- GOOD -->
div {
border: 1px solid #0000;
}
<!-- BAD -->
div {
border-width: 1px;
border-style: solid;
border-color: #000;
}
- Use CSS preprocessors. With only basic CSS web developers cannot reuse a set of rules in several selectors that contain unclear data across a stylesheet. A CSS preprocessor provides a sophisticated approach to writing that extends CSS functionality and allows generation of code from the program’s unique syntax. This advanced code is compiled into standard CSS code that the browser can comprehend. The 4 most popular CSS preprocessors are Sass, LESS, Stylus, and PostCSS.
- Use
media
queries. Using media queries, you can apply CSS styles based on the overall kind of device (print vs. screen) or other attributes (screen resolution, browser viewport width, etc.). - CSS minification. When utilized excessively, large CSS files might make your site load more slowly and drive users away. Minification speeds up the browser's download and processing of these files, enhancing user experience and page performance by removing superfluous data from the CSS code.
JavaScript
<script></script>
tag positioning. In an HTML document, you can include the<script></script>
tag inside the head part or the<body></body>
section. Nevertheless, as it might speed up a webpage's load time, adding scripts to the bottom of the<body></body>
element is one of the best web development practices in the front end. Because the browser won't allow any other content to render until the script is run, placing the script tag at the beginning of the HTML document will cause the website to load more slowly.
<!DOCTYPE html>
<html>
<head>
<title>My Webpage</title>
</head>
<body>
<!-- Your HTML content here -->
<script type="text/javascript">
// Your JavaScript code here
</script>
</body>
</html>
- Use
<noscript></noscript>
tag. When a user disables scripting in their browser, the<noscript></noscript>
element in HTML is used to show text for those browsers that do not support script tags. The <head> and <body> tags both can include this tag. - Minify your JavaScript code. Just like with CSS file minification, this practice means eliminating all superfluous characters from JavaScript source code for smaller file sizes without compromising its functionality. It entails using shorter variable names and functions and eliminating semicolons, whitespace, and comments.
/* Original code*/
let tabs = this.querySelectorAll('.tab_header');
let tabTarget = tab.querySelector('.tab_header')
if (window.innerWidth < 800) {
if (tabTarget.classList.contains('active')) {
tabTarget.classList.remove('active');
} else {
this.toggleClasses(tabs, tabTarget);
}
} else {
this.toggleClasses(tabs, tabTarget);
}
/*Minified code*/
let tabs=this.querySelectorAll(".tab_header"),tabTarget=tab.querySelector(".tab_header");window.innerWidth<800&amp;&amp;tabTarget.classList.contains("active")?tabTarget.classList.remove("active"):this.toggleClasses(tabs,tabTarget);
- Avoid inline JavaScript in HTML. Integrating JavaScript directly into your HTML pages is not regarded as a recommended approach. Avoiding inline JavaScript is one of the web development best practices aimed at enhancing maintainability and keeping the presentation layer and controller layer separate. As the project grows in scope, it will become more challenging for you and others to manage if there is inline JavaScript on multiple pages.
Back-end web development best practices
Here, you can also find the acknowledged strategies and methods for server-side programming and architecture useful for all devs.
- Opt for a distributed architecture. By dividing your workload over several servers, a distributed architecture may handle traffic more effectively than a single server. A distributed system is a group of computer programs that work together to accomplish a common objective by sharing computational resources among several distinct computation nodes. The goal of distributed systems is to rid a system of inefficiencies and primary centers of failure.
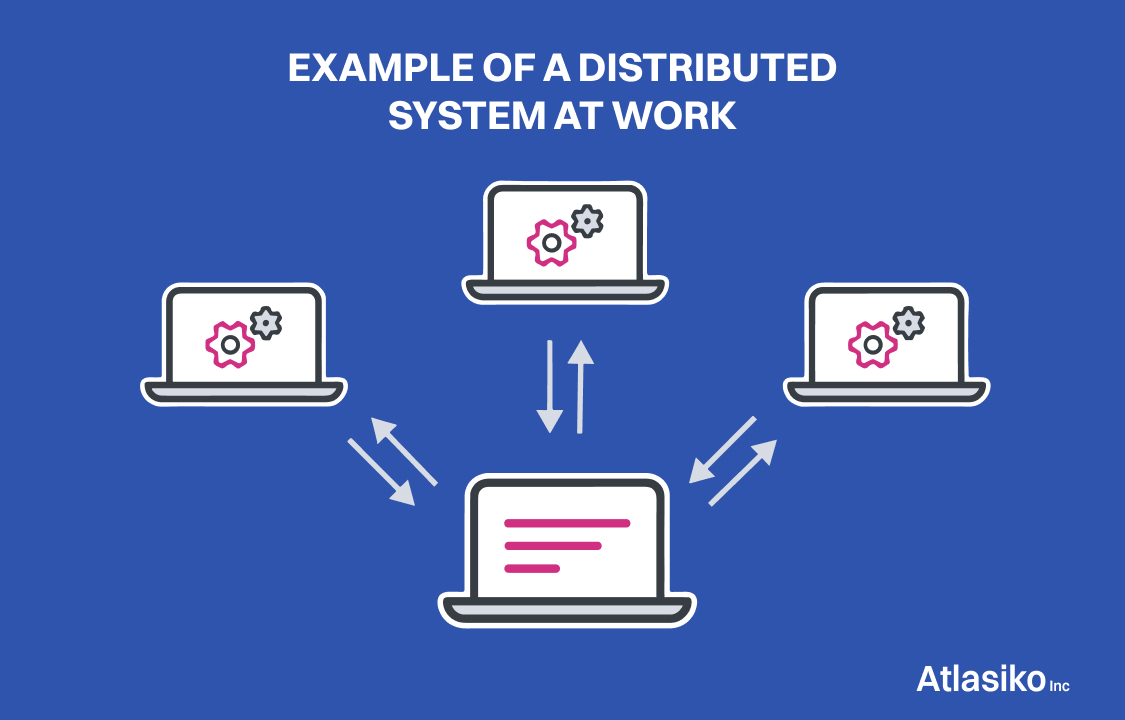
- Optimization of database queries. Database queries can have a big effect on how well your system works. Many NoSQL and graph databases, as well as traditional database management systems, include query optimization built in. Database sharding, query caching, and indexing can all be used to improve database queries. The query optimizer looks at several query plans to find the most effective way to run a particular query.
- Enabled caching. Use caching to enhance response speeds and lower database load. To cache static files, use caching techniques like content delivery networks (CDNs) or in-memory caching (Redis or Memcached). Depending on the needs of your web application, implement caching at various levels, such as query caching, full-page caching, or application-level caching.
- Horizontal scaling. To manage greater traffic, you can expand your system's server count through horizontal scaling as one of the best web development practices for the back end. Horizontal scalability can be achieved with containerization technologies such as Docker and Kubernetes.
- Use a separation of concerns principle. One of the best strategies for developers and the most crucial components of architecture is the separation of concerns. Businesses employ this strategy to accurately divide security issues and enhance code maintainability. Using this method, back-end developers can modularize their code.
- Opt for REST API architecture. This approach to a distributed API design is considered the most popular practice in back-end development. It is based on HTTP protocol and utilizes HTTP verbs to carry out CRUD (create, read, update, and delete) functions on resources. It’s important for back-end developers to completely understand and master this style since many consider REST a de facto standard of web architecture.
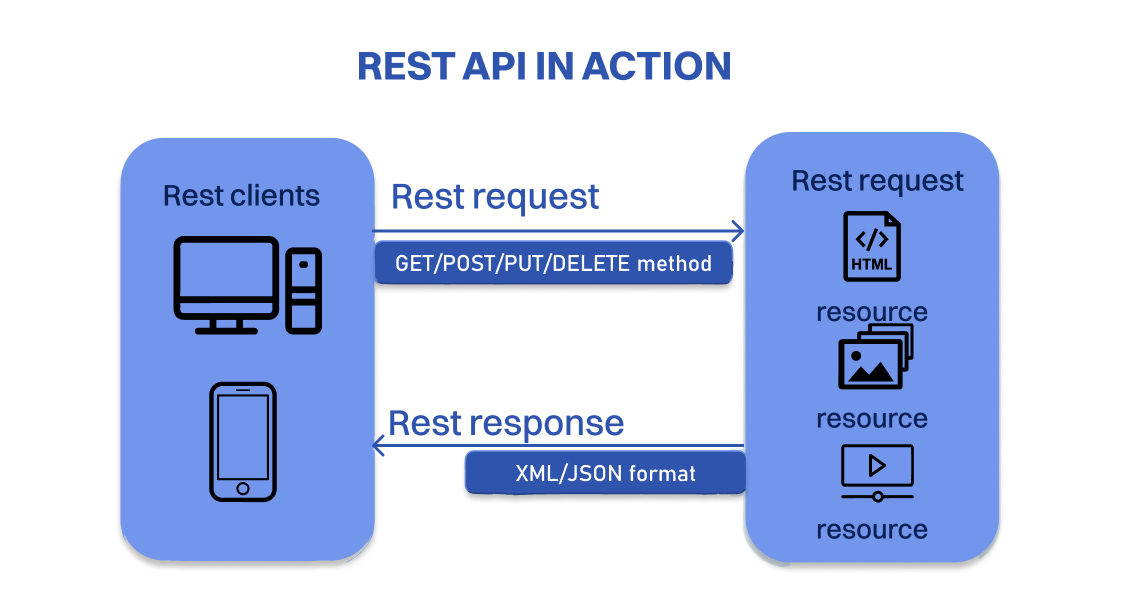
- Microservices architecture for scalable code. Your system can be divided into smaller, standalone functions that can be designed and implemented independently thanks to microservices architecture. This method makes it simpler to scale individual services as needed, which can increase the scalability and dependability of your system.
- Proper error handling. Put in place thorough error handling procedures to handle exceptions with grace and give clients insightful error messages. It is the procedure for precisely anticipating, identifying and fixing bugs in web programs, applications, or communications. Debugging and troubleshooting are made easier by logging errors and exceptions.
- Automated testing. Website development best practices include using automated testing techniques for developing back-end applications. Use integration testing to make sure different components communicate with each other smoothly. Building, testing, and deployment procedures can be automated with the help of CI/CD pipelines that are a part of DevOps methodology, guaranteeing a dependable and stable back-end.
- Use efficient version control systems. To keep track of changes in the back-end codebase, developers employ version control tools such as Git. Create efficient branching plans for team development, and preserve the quality of your code by doing regular code reviews.
- Monitor and analyze performance. Professionals use monitoring tools to keep tabs on back-end systems' health and performance. To track server metrics, response times, and resource usage, you can choose programs like Prometheus, Grafana, Nagios, Zabbix, or New Relic. To maintain optimal system performance and proactively resolve performance concerns, devs set up alerts and notifications.
Best practices for web development from our experts
Here are some practical tips that our professional developers would like to share with you. These are some of the fundamental principles they adhere to while working on your projects to ensure meticulous execution and successful results.
We offer impeccable expertise and a wide range of efficient solutions for your business development
Be consistent in planning
Often, developers bypass the initial setup process and jump straight into the coding, which results in having to redo work - a process that takes up precious time. To avoid this, it's key to first outline the bigger picture and set up a definite objective for your project. Charting a course towards this primary goal doesn't just save resources and energy. It also fosters comprehensive and precise creation, spotlighting essential project elements and milestones.
Keep business goals in mind
To produce the most satisfying results, you need to keep in mind not only the project’s objectives but general business goals of the organization you make that solution for. Otherwise, if the outcomes of your work don’t meet client expectations and principles, it may turn out a complete failure. Ensuring the alignment of your digital product with the client’s organizational values is one of the primary requirements for professional developers as well as one of the best practices for web development.
Choose the right tech stack
Using the best technologies for web development is the way to implement all features and functionality planned for your solution as well as ensure its proper performance. Employ the best web development practices for front-end and back-end development we mentioned above, expand your skills in programming languages, and learn how to work with different web development frameworks. Our professionals in web development always work only with the use of the most advanced technologies.
Ensure design responsiveness
Nowadays, responsive design is considered to be one of the main web development trends requiring an application or website's design to adjust to different kinds of devices. People use different devices with different screen sizes to view content, so it's vital to have this feature. Having a responsive design also boosts a website's visibility on search engines so we recommend adhering to this practice during web development.
Use robust CMS
Take advantage of pre-made modules and templates available through content management systems (CMS) and tools such as WordPress, GudHub, Joomla, Wix, HubSpot CMS, or others. When there is a tight deadline, these tools can come in handy. Choose the framework or tool that best suits your level of experience and the demands of the project.
Emphasize security
People today value data safety. They study data and website requirements closely to ensure their information is safe. That’s why adding extra security layers is one of the web application development best practices. Showing that you're serious about protecting important data can help gain trust from users.
Write simple and smart code
The longer the code, the more likely it is to have errors, which will negatively impact the speed of your website. The secret to creating creative programs that minimize needless errors is to make sure each code addresses an issue or has a specific aim, like adding a feature to a certain website page. When writing smart codes, consider providing code comments as well.
Ensure cross-platform and -browser compatibility
Ensure that your content and functionality are shown and work appropriately on all platforms and browsers for a high-quality user experience. Even the slightest differences in hardware and software settings can have a significant influence on the end-user experience. To ensure compatibility run cross-platform and cross-browser tests. All of these procedures are together referred to as "compatibility testing." Ideally, the more devices you use, the better coverage your product should be able to attain.
Run tests regularly
Undoubtedly, regular testing is one of the development best practices that every expert web developer has to follow. During testing, make sure you write clean, readable code by using best practices and tools for web development to ensure a smooth testing procedure. Before letting your clients use your web app or website, you have to make sure it works well and is free of bugs. Remember that the total rating of your web solution is heavily influenced by testing and quality assurance.
Monitor performance and optimize
It's also crucial to monitor how your web-based solution reacts to traffic and assess whether it can manage the expected level of requests. For efficient performance monitoring and optimization, you can use tools like GTmetrix, and PageSpeed Insights. All these tools are available to thoroughly inspect websites and provide you with an extensive report. Based on this analysis, web developers correct the issues and take measures to prevent them from happening again.
Consider SEO
Among the best practices in web development, we can note the focus shift toward greater consideration of search engine optimization. For many website creation projects, employing SEO web development is a requirement, so be sure to learn its methods and characteristics.
Conclusion
We hope this article gave you more insights into the best practices website development employs for achieving guaranteed high-quality results. Even though new technologies in this field emerge every day, you can always rely on time-tested methods and proven basics to facilitate the development process. To discover more useful information, follow our web development blog.